- Qt Disconnect All Slots
- Qt Disconnect All Slots From Signal To Iphone
- Qt Disconnect Signal
- Qt Connect Signal Slot
- Qt Signals And Slots Example
- Qt Disconnect All Slots From Signal Switch
- Qt Disconnect All Slots From Signal Box
- Disconnect specific slot from all signals. Now I want a function to disconnect all the signals from receiver’s slot. Then all of the signals/slots.
- In this article, we will explore the mechanisms powering the Qt queued connections. Summary from Part 1. In the first part, we saw that signals are just simple functions, whose body is generated by moc.They are just calling QMetaObject::activate, with an array of pointers to arguments on the stack.Here is the code of a signal, as generated by moc: (from part 1).
- Apr 13, 2016 Signals and slots are the basic foundation of Qt C GUI Application. In this QT tutorial we will learn signal and slots tutorial fnctions work by creating an example application.
May 30, 2016 In this tutorial we will learn How to use signal and slots in qt.How Qt Signals and Slots Work. Understanding Signals and Slot in Qt. Qt Tutorials For Beginners – Qt Signal and slots May 30, 2016 admin C, Qt 2. Disconnect (ui- horizontalSlider, SIGNAL (valueChanged (int)).
One key and distinctive feature of Qt framework is the use of signals and slots to connect widgets and related actions. But as powerful the feature is, it may look compelling to a lot of developers not used to such a model, and it may take some time at the beginning to get used to understand how to use signals and slots properly. However, since version 4.4, we can relay on auto-connections to simplify using this feature.Back in the old days, signals and slots connections were set up for compile time (or even run time) manually, where developers used the following sentence:
this is, we stated the sender object's name, the signal we want to connect, the receiver object's name and the slot to connect the signal to.
Now there's an automatic way to connect signals and slots by means of QMetaObject's ability to make connections between signals and suitably-named slots. And that's the key: if we use an appropriate naming convention, signals and slots will be properly connected without the need to write additional code for that to happen. So by declaring and implementing a slot with a name that follows the following convention:
uic (the User Interface Compiler of Qt) will automatically generate code in the dialog's setupUi() function to connect button's signal with dialog's slot.
So back to our example, the class implementing the slot must define it like this:
We then write the method's implementatio to carry on an action when the signal is emitted:
In brief, we have seen that by using automatic connection of signals and slots we can count on both a standard naming convention and at the same time an explicit interface for designers to embrace. If the proper source code implements such a given interface, interface designers can later check that everything is working fine without the need to code.
Qt Disconnect All Slots
I have an old codebase I started writing using the Qt 3.x framework—a little while before Qt4 was released. It’s still alive! I still work on it, keeping up-to-date with Qt and C++ as much as possible, and I still ship the product. Over the years I have moved the codebase along through Qt4 to Qt5, and on to compilers supporting C++11. One of the things I’ve sometimes found a little excessive is declaring slots for things that are super short and won’t be reused.
Here’s a simplified example from my older code that changes the title when a project is “dirty” (needs to be saved):
2 4 6 8 10 12 14 16 18 20 22 | classmyMainWindow... privateslots: void_setTitle(constQString&inTitle ); // implementation connect(inProject,SIGNAL(signalDirtyChange(bool)), ... voidmyMainWindow::slotProjectDirtyChanged() QString name=mProject->name(); name+='*'; _setTitle(name); |
(Aside: If you are wondering about my naming convention, I developed a style when using Qt early on (c. 2000) where signals are named signalFoo() and slots are named slotFoo(). This way I know at a glance what the intent is. If I’m about to modify a slot function I might take an extra minute to look around since most IDEs can’t tell syntactically where it’s used in a SLOT()
macro. In this case you have to search for it textually.)
New zealand gambling law guide. New Zealand Gambling Law Guide. Home; Table of Contents; Recent Updates; Subscribe; New Zealand Gambling Law Guide. Keyword Search Go Navigation. Table of Contents; Gambling Act Sections; Gambling Act Regulations; 2016 Game Rules; Gambling Commission Decisions; Gambling Statistics and Information; Awards; About Us.
Thanks to C++11 lambdas and Qt’s ongoing evolution, these short slots can be replaced by a more succinct syntax. This avoids having to declare a method in your class declaration and shortens your implementation code. Both desirable goals!
Let’s take a look.
Getting rid of SIGNAL() and SLOT() macros
The first really nice thing about Qt5 is that we can move away from the classic SIGNAL()
and SLOT()
macros to using method pointers.
This does several things for us:
- relieves us of having to lookup and fill in the parameters for the methods
- shortens your code
- IDEs can identify the methods used in a connect call when you search for uses of a method
- allows implicit conversion of arguments
- catches problems at compile time rather than runtime
This last one is the most important. If the two methods you are trying to connect are mismatched it simply won’t compile. I can say with authority that problems found at compile time can save you many, many hours of debugging!
So if we apply this idea to our example, the connect()
call becomes:
2 | connect(inProject,&myProject::signalDirtyChange, [=] () { } ); |
Here we connect our signal to an anonymous function which has access to the variables in the current scope. We leave out the return type so void
is inferred.
(This is a very quick intro to lambdas. You can find a much more in-depth description at cppreference.com and in this post.)
Our final result
Here’s our result if we put all that together. We got rid of the extra declaration in the header and the extra function in the implementation. Much nicer.
2 4 6 8 10 12 14 16 | classmyMainWindow... private: ... connect(inProject,&myProject::signalDirtyChange, [=] () { if(mProject->dirty()) }); |
What about the arguments?
As I mentioned earlier, lambdas can also take arguments, so you can pass the parameters from the signal into the lambda function. For example, I recently ran into an issue where I needed to accept a QRectF
, adjust it, and send it on again. I did it like this:
Qt Connect Signal Slot
2 4 | connect(mNavView,&myNavView::signalNavBoxChange, [this] ( const QRectF &inRect ) { const QRectF cAdjusted( inRect.topLeft() / mRatio, inRect.bottomRight() / mRatio ); mView->slotMoveView(cAdjusted); |
I’m not aware of any advantages of one over the other – except that maybe reducing the scope the lambda function body has access to may be desirable. If you have any opinions on this, please leave a comment!
Caveat
Depending on how you work, using lambdas may make testing more difficult. Having separate methods you can call in testing might be more desirable.
Qt Signals And Slots Example
It’s also tempting to use lambdas in lots of places. It’s pretty powerful. I think, however, that restricting it to simple things like the above—replacing short one-use slots, adjusting data before passing it along, or for simple callbacks—will be the most maintainable in the long run. Until we work with them and live with the resulting code for a few years we won’t know…
Hope that helps someone out there on the information superhighway!
* Lambdas Using References
Qt Disconnect All Slots From Signal Switch
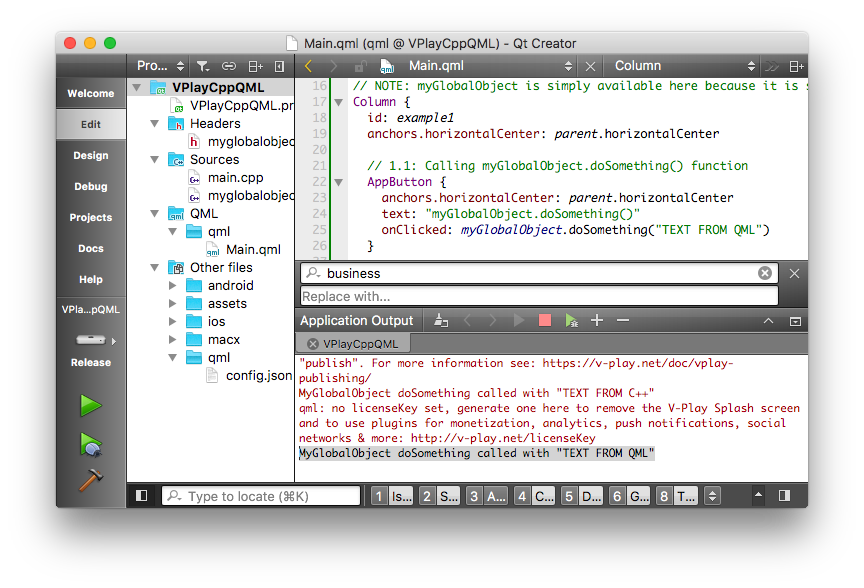
Qt Disconnect All Slots From Signal Box
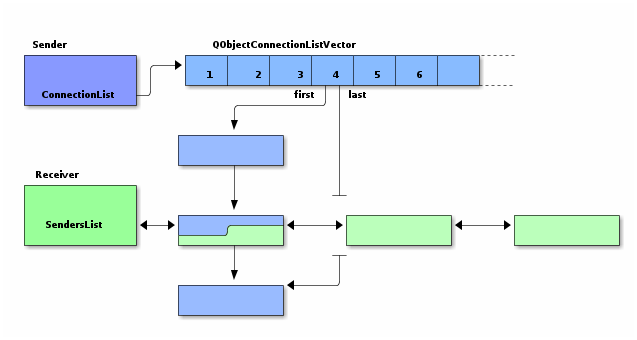
As Ronaldo Nazarea points out in the comments:
You have to be careful though about capture by reference (or pointers if by value). If the slot is not run inside the scope of the definition, you may end up with references to invalid locations caused by out of scope variables.